Table of contents
Introduction
This tutorial will teach you how to get the table of contents information from a publication. After the tutorial you will know how to render the table of contents as an HTML list and make the reading system navigate to a chapter when you click on a chapter title.
Audience
Typescript developers.
Prerequisites
Full code example
For reference, you can find the full code example in the Web framework tutorial repository.
In order to set up the developer environment including where to put your credentials, please follow the steps described in Set up tutorial environment.
Tutorial
The code in this tutorial builds upon the code from the Basic Navigation tutorial.
Create table of contents class
First, create a class responsible for rendering the table of contents in a new file, AppTableOfContents.ts
.
export class AppTableOfContents {
}
As it does not need to have a state, all functions will be static.
Get table of contents from publication
The first time a ReaderPublication is asked for the navigation information the ReaderPublication fetches and parses the resources containing the navigation information.
The publication navigation can contain many types of navigation collections, like landmarks, list of illustrations and table of contents. To build a table of contents you only need the TOC navigation collection.
static async create(readerPublication: IReaderPublication, readerView: IReaderView, tocContainer: HTMLElement): Promise<void> {
tocContainer.innerHTML = "";
const publicationNavigation = await readerPublication.fetchPublicationNavigation();
const tocCollection = publicationNavigation.getNavigationCollections()
.find(collection => collection.getType() === NavigationCollectionType.TOC);
if (tocCollection) {
const tocListElem = AppTableOfContents.renderTocListElement(tocCollection.getChildren(), readerView);
tocContainer.append(tocListElem);
}
}
Render the table of contents
The table of contents navigation collection
The table of contents (TOC) navigation collection consists of zero or more navigation items. The text content of a navigation item in the TOC is almost always a chapter title.
navigationItem.getTextContent();
The TOC navigation collection is a tree of navigation items. This means that each navigation item may have child items that you can access using navigationItem.getChildren()
.
Render the elements
In this tutorial you will render the table of contents as an ordered list, but feel free to experiment and make the table of contents look and feel as you want.
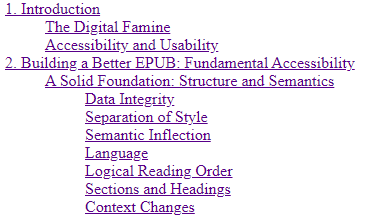
Table of contents tree structure
To render the collection, create an ordered list HTML element and add each rendered navigation item to the list element. Pass the ReaderView to the method that renders the links, to have a way to navigate to the position given by the navigation item.
private static renderTocListElement(
navigationItems: ReadonlyArray<IReaderPublicationNavigationItem>,
readerView: IReaderView
): HTMLOListElement {
const itemElements: HTMLLIElement[] = navigationItems.map(item => AppTableOfContents.renderTocItemElement(item, readerView));
const listElem: HTMLOListElement = document.createElement("ol");
listElem.append(...itemElements);
return listElem;
}
Each navigation item is represented by an <li>
element. If there is a locator, render an <a>
element that navigates to the chapter. The navigation item may also contain a list of children, for example when a chapter is divided into subchapters. Let’s reuse the renderTocListElement
method to render the children.
private static renderTocItemElement(navigationItem: IReaderPublicationNavigationItem, readerView: IReaderView): HTMLLIElement {
const listItemElem: HTMLLIElement = document.createElement("li");
// Create an anchor element to make the item clickable
const chapterAnchor: HTMLAnchorElement = AppTableOfContents.renderTocItemAnchorElement(
navigationItem,
readerView
);
listItemElem.appendChild(chapterAnchor);
// Recursively render any subchapters
const children = navigationItem.getChildren();
if (children.length > 0) {
const childListElem = AppTableOfContents.renderTocListElement(children, readerView);
listItemElem.appendChild(childListElem);
}
return listItemElem;
}
For each navigation item that has a locator, render an <a>
element. Replace the normal click handling with a function that tells the reader view to navigate to the correct part of the publication.
private static renderTocItemAnchorElement(navigationItem: IReaderPublicationNavigationItem, readerView: IReaderView): HTMLAnchorElement {
const anchorElem = document.createElement("a");
anchorElem.textContent = navigationItem.getTextContent();
anchorElem.href = '';
anchorElem.addEventListener('click', (ev) => {
ev.preventDefault();
// Tell the ReaderView to navigate to the position given by the navigation item
readerView.goTo(navigationItem)
.catch(_ => Logger.logError("Failed to navigate to chapter " + navigationItem.getTextContent()));
});
return anchorElem;
}
Integrating with the App
index.html
Right after the readingsystem-viewport
<div>
, add another container element for the Table of contents.
<div id="table-of-contents"></div>
App.ts
Add the table of contents element as a parameter to the constructor of the App. Each time you load a new EPUB publication the App renders a new table of contents to this element.
constructor(
viewElement: HTMLElement,
private tableOfContentsElement: HTMLElement,
licenseApiKey: string,
private userId: string) {
// ...
}
Add a call to render the table of contents in the end of the App’s loadEpub
function.
await AppTableOfContents.create(
this.readerPublication,
this.readerView,
this.tableOfContentsElement
);
index.ts
Pass the table of contents element to the App
constructor.
const tableOfContentsElement = document.getElementById('table-of-contents');
if (viewElement && tableOfContentsElement){
const app = new App(viewElement, tableOfContentsElement, apiKey, loggedInUserId);
}
index.css
Because some publications already have chapter numbers in the text provided in the table of contents navigation collection, remove the default list item numbers from the css.
#table-of-contents li {
list-style: none;
}
Conclusion
That’s it. You should now have an app set up with a clickable table of contents that navigates you to the correct part of the publication.
Concepts
Locator
Used to reference locations, both single point and ranges, in a publication. Can be serialized to format specific to the type of publication.
Navigation collection
A collection of navigation items. For example: landmarks, list of illustrations, and table of contents.
Navigation item
An item containing text and a location to navigate to. Might have more navigation items as children.
ReaderPublication
A version of a publication processed to be rendered in a ReaderView.
ReaderView
Handles rendering and navigation.